- Jinja2 templating tutorial.
- The tutorial can be found here, https://realpython.com/blog/python/primer-on-jinja-templating/.
- Flask is using Jinja2 as its templating engine.
- The cool thing about Jinja2 is that it is using .html extension.
- The command to install Jinja2 is
sudo pip3 install jinja2
or if in virtual environment the command will be pip3 install jinja2
.
- Anyway, if you install Flask from
pip
it would also install Jinja2 automatically.
- Jinja2 is part of
from flask import render_template
.
- You can put any arbitrary variables in the HTML template using
render_template()
function. This is an example.
@app.route("/")
def index():
render_template(
"which_template_you_want_to_use.html",
any_variable_1="any_value_although_it_is_usually_string",
any_variable_2="any_value_although_it_is_usually_string",
any_variable_3="any_value_although_it_is_usually_string",
any_variable_4="any_value_although_it_is_usually_string",
any_variable_5="any_value_although_it_is_usually_string"
)
- Then these variables can be called in the template
"which_template_you_want_to_use.html"
with {{any_variable_1}}
.
- This is an example specifically for Flask.
- There is template inheritance as well.
- The sub - template is what usually the main display as it is the same case with
which_template_you_want_to_use.html
.
- The super template is the main template (the larger HTML files with all CSS and JS files attached into it) that will be added with functionalities from the sub - template.
- So in analogous, super template is the function and the sub - template is the arguments.
- But needed to be known that argument can be a function as well.
- Hence, this can be further dumbed down into several sub - template.
- There is block tag like this,
{% block %}
this is meant for the sub - template to fill and for super template to reference which part of it got filled by child template.
- Example
{% block %}
in sub - template.
{% block this_from_sub_template %}
{# Put some HTML or Jinja2 codes here. #}
{% endblock %}
- Example
{% block %}
in super template.
{% block this_from_sub_template %}
{% endblock %}
- In super template we just do not fill anything in between of the Jinja2 tag.
- Do not forget to put
{% extends "the_super_template.html" %}
in the uppermost of the sub - template.
- If you need to render a block from super template then use this
{{super()}}
. This is the example codes.
{# These codes are in sub - function. #}
{% block footer %}
{# These codes will take `footer` block from whatever this template extends from. #}
{{super()}}
{% endblock %}
- There is macros as well in Jinja2.
- Macros is like a function but for the templating engine.
- Here is an example of macros in Jinja2.
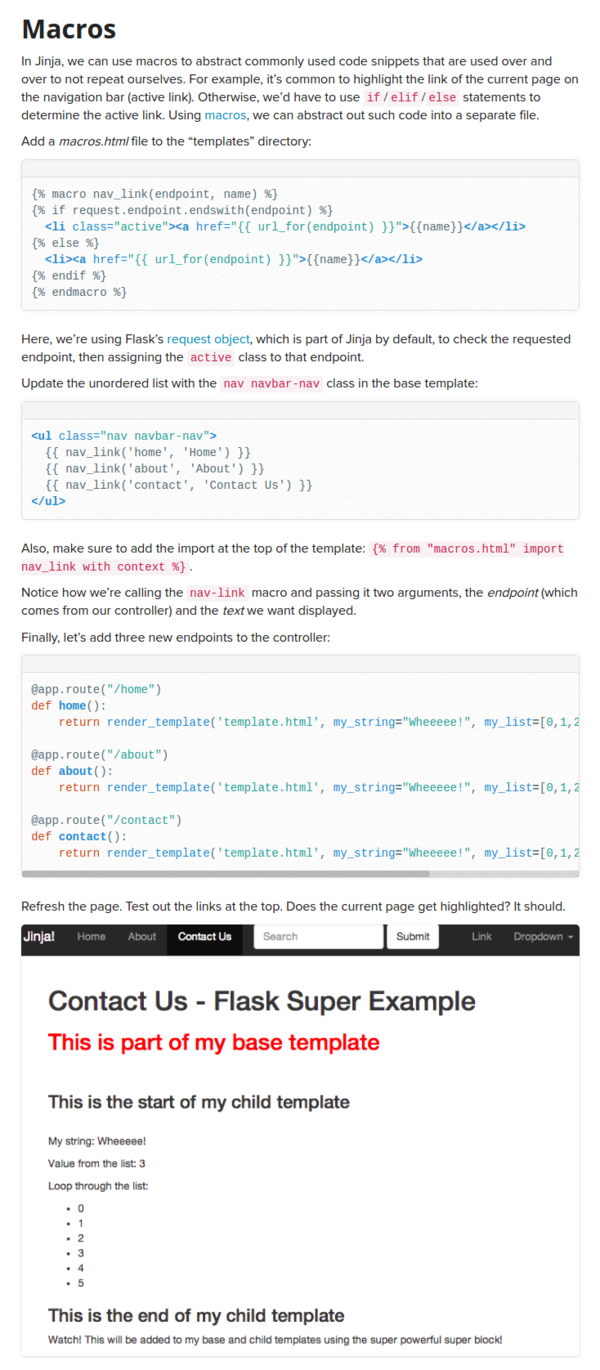
- Here is an example of using built - in filter as well as to make custom filter.
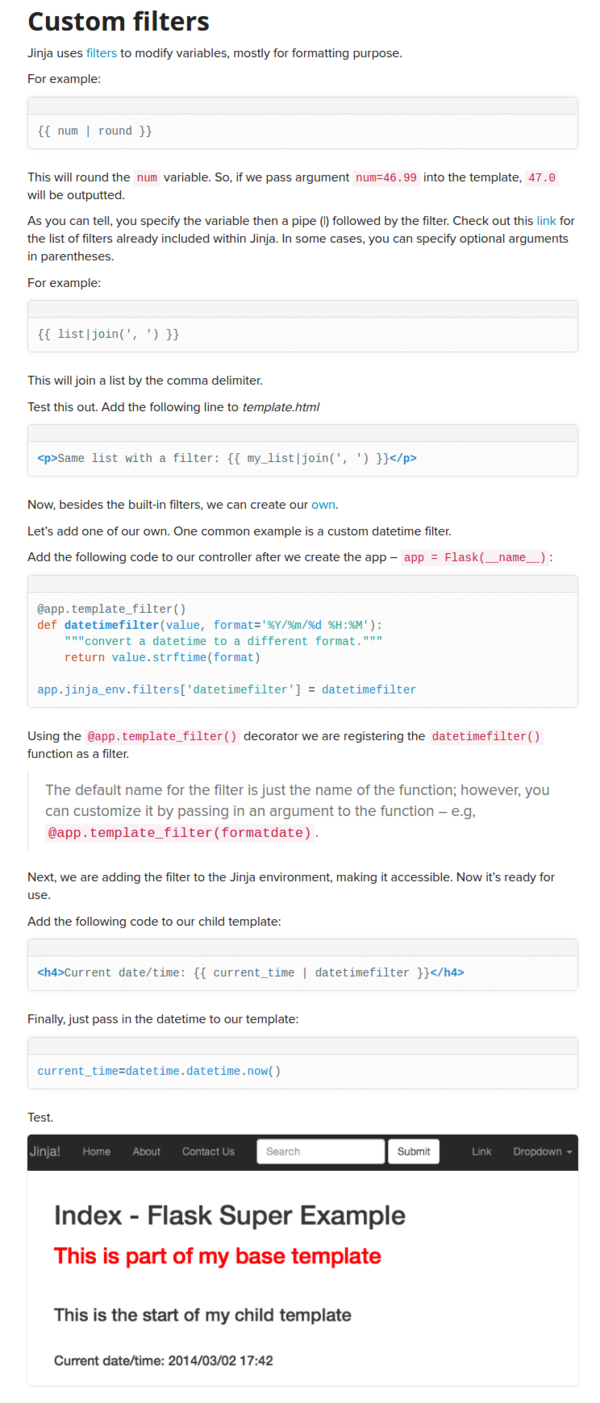
- Here is the screenshot of the tutorial.

- Here is all kind of expression that can be used within Jinja2.
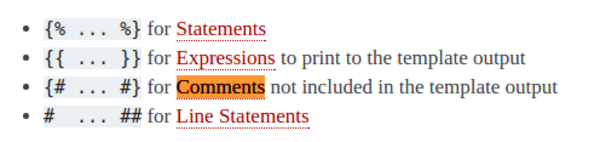