- What does
using namespace
means?
- I found the answer in this link, http://www.cplusplus.com/forum/beginner/49748/.
- Usually
using namespace
is used for using namespace std;
. std
is the standard library for C++. std
has the basic operation like cout
, cin
, ....
- However, if we are using multiple libraries there could be multiple functions with same name.
- Let say library
std
and dummy
, each, has a function called dummy_function()
. Without using namespace std;
means there are two definitions of dummy_function()
. Usually without using namespace std
the proper function call will be std::dummy_function()
or dummy::dummy_function()
.
using namespace std;
means to always use function from std
library if there are duplicate names. This means with only writing dummy_function()
the compiler will always take dummy_function()
from std
instead of dummy
.
- Below is the screenshot from the discussion about
using namespace std;
means.
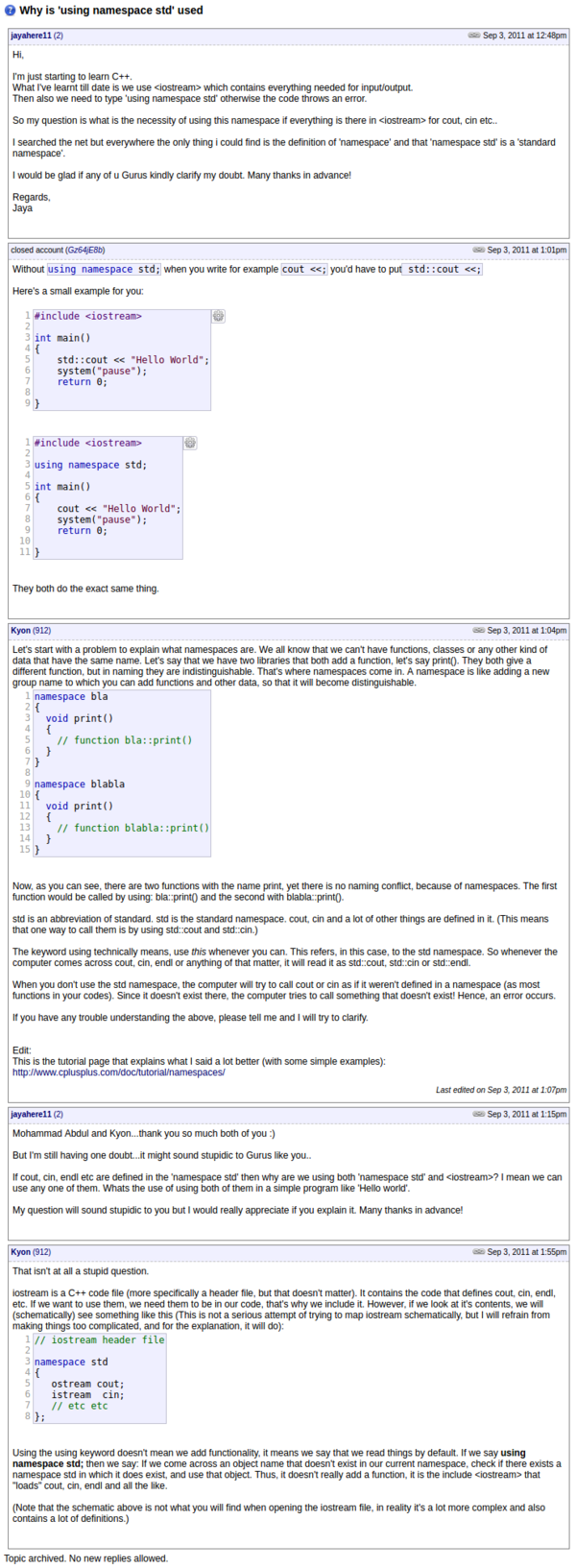
./20170807-1503-cet-1-2.png
- What does
return 0;
means?
- The
0
refer to the error value.
- The
int
in int main()
is because in the main runtime C++ codes return an integer or error codes.
- This is very useful when executing multiple commands in terminal.
- What is the different between /usr/lib and /usr/local/lib?
- /usr/lib is used for library installed by operating system.
- /usr/local/lib is used for library compiled and then installed by operating system.
- Every
class
declaration in C++ followed with semi - colon.
- What is
namespace
?
- There were often mistakes, I wrote this codes
g++ -o -v output_file main.cpp
. Whereas the correct compilation codes is like this, g++ -o output_file -v main.cpp
.
- Make sure to put appropriate arguments for each parameters! In this case
-o
should always be followed with the name of the output file.
- There is this term called "function prototype". After searching, it is actually a normal function but without the content. Prototype function only mentions what are the inputs and what is the output.
- In C/C++ usually semicolon ";" is put in the end of the function declaration.
- Access specifiers are a notion on the accessibilities of a function or variable. The most common access specifiers are public, private, and protected.
- Public functions or variables can be accessed from anywhere within the same program.
- Private functions or variables can be accessed within same class or a friend functions.
- What is friend function? Friend function is a function that can access property of other private function. There are two kinds of friend function.
- Globally defined friend function.
- Bidirectional friend function.
- I have a question about why inheritance is always followed by public statement. This is the example.
class foo;
class bar:public foo;
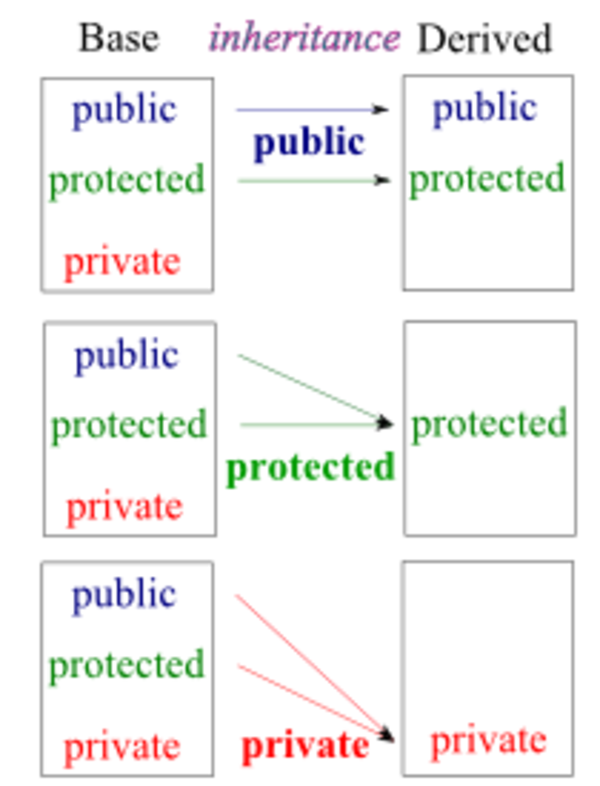
./20170807-1503-cet-1-4.png
- Basically private variable in parent cannot be inherited unless it is a friend function or variable.
- For publicly inherited class the public and protected function and variable are keep into their respective access specifier in the sub - class.
- For private inherited class the public and protected function and variable are converted into private in the sub - class.
- For protected inherited class the public and protected function and variable are converted into protected in the sub - class.
- This is the screenshot of the article.

./20170807-1503-cet-1-6.png
- Function prototype is like a strict blueprint that need to be followed. Here is an example of function prototype.
void test_prototype_function(int _i);
- The strict is to ensure that when the function is created it needs to follow that the specification provided from the function's blueprint.
- Usually blueprints is placed in a header file.
- So, header file .h is used to provide blueprints while the .cpp is used to provide definitions and implementations part.
- I was wondering what part of codes should be in header file and which part of codes should be in the .cpp file. I found this .pdf with quite explanations.
./20170807-1503-cet-1-7.pdf
- Some that I understand from the .pdf.
- In header file there need to be a safety guard. Examples of safety guards are
#pragma once
and #ifndef
followed with #define
, the content of the library, and then #endif
.
- The declarations! Everything that has value and take space in runtime or static does not belong to the header file.
- Set up global variable that can be used for the entire program to be declared with, for example,
extern int sample_integer
. Then later in the .cpp file define that variable with, for example, int sample_integer = 0;
. I think extern
is only for C and not C++ in particular.
- Every .h file should include every other library dependencies.
- There is this example about using
struct
. Basically if I need to create incomplete/forward declaration. Then keep declare the same struct
later as a normal variable. The thing here is to have incomplete/forward declaration first before use it later in the same header .h file.
- Based from a discussion here, https://softwareengineering.stackexchange.com/questions/167723/what-should-and-what-shouldnt-be-in-a-header-file. Every statements that generates data and code as they parsed does not belong in the header (.h) file.
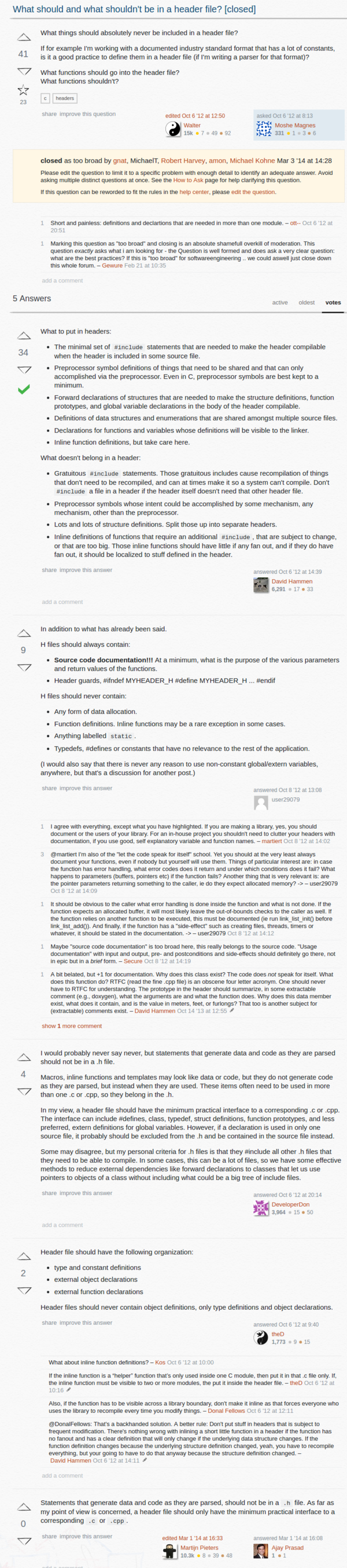
./20170807-1503-cet-1-9.png
protected
access specifier is a little bit weird because it prevent the class itself to access the protected functions or variables. However, protected
allows the functions or variables to be accessed in the children/inherited classes.
- Reference for protected class, is in this link, https://msdn.microsoft.com/en-us/library/e761de5s.aspx.
- In my own example, protected class is like when I have a class named
human
and it inherits into arm
, body
, head
, leg
, .... The body has a protected
function called reduce_hit_point()
but can only be accessed from the children classes. Hence, this way there is no way the HP (hit point) reduced from the human
class but the children classes.
virtual
is a member function that let the children classes to override/polymorph a function (or variables).
- If
virtual
is not used the function is not polymorphed despite there is a same name function in the child class (the original function from parent is the one who still be called).
- Here is a documentation about
virtual
, https://msdn.microsoft.com/en-us/library/0y01k918.aspx.
- When to use
float
and when to use double
?
- In many cases C++ developer would use
double
over float
.
float
only be used when dealing with a thousands of float
values without concern on accuracy.
- A discussion here, https://softwareengineering.stackexchange.com/questions/188721/when-do-you-use-float-and-when-do-you-use-double, mentioned that "If you have an input of 100,000 numbers from a file or a stream and need to sort them, put the numbers in a
float[]
."
- Here is the screenshot of the discussion.

./20170807-1503-cet-1-11.png
- Here is an example of selective friend using Attorney - Client relationship.
class problem {
private:
friend class attorney;
void hello_1();
void hello_2();
void hello_3();
int i;
};
class attorney {
private:
friend class client_2;
static void hello_1 (problem& _p){ _p.hello_1(); }
static void hello_2 (problem& _p){ _p.hello_2(); }
};
class client_1 {
problem p;
attorney::hello_1(p); // Error.
attorney::hello_2(p); // Error.
};
class client_2 {
problem p;
attorney::hello_1(p);
attorney::hello_2(p);
};
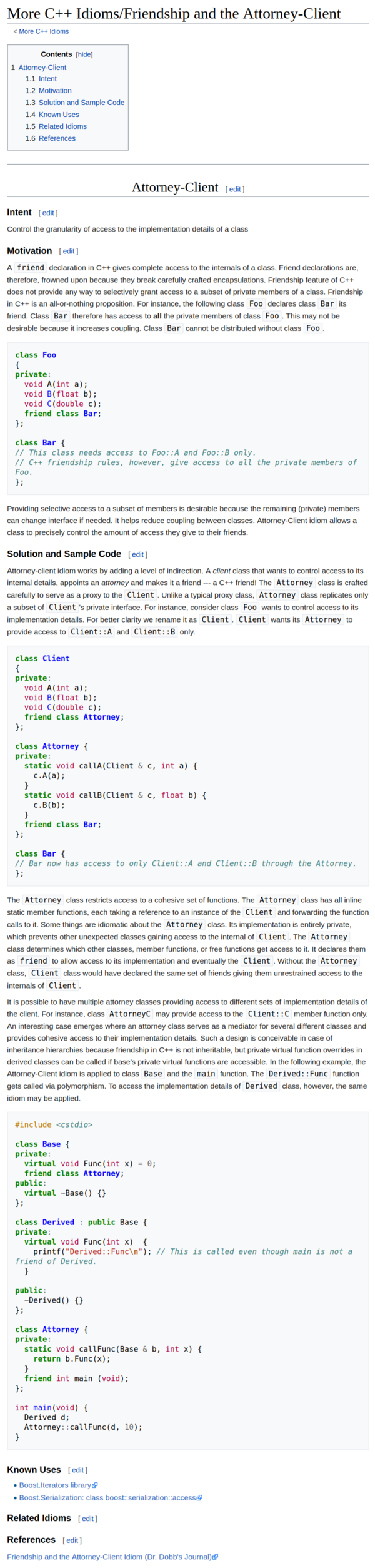
./20170807-1503-cet-1-13.png

./20170807-1503-cet-1-15.png
- In the nutshell.
public
means the everything program - wide can access the function or the variable.
private
means only the class object can access the function or the variable.
protected
means only the children or friend class can access the function or the variable (the class object itself cannot access protected variable).
- Here is a tutorial on how to install QT Studio in Ubuntu, https://wiki.qt.io/Install_Qt_5_on_Ubuntu.
- Here is the screenshot of the tutorial.
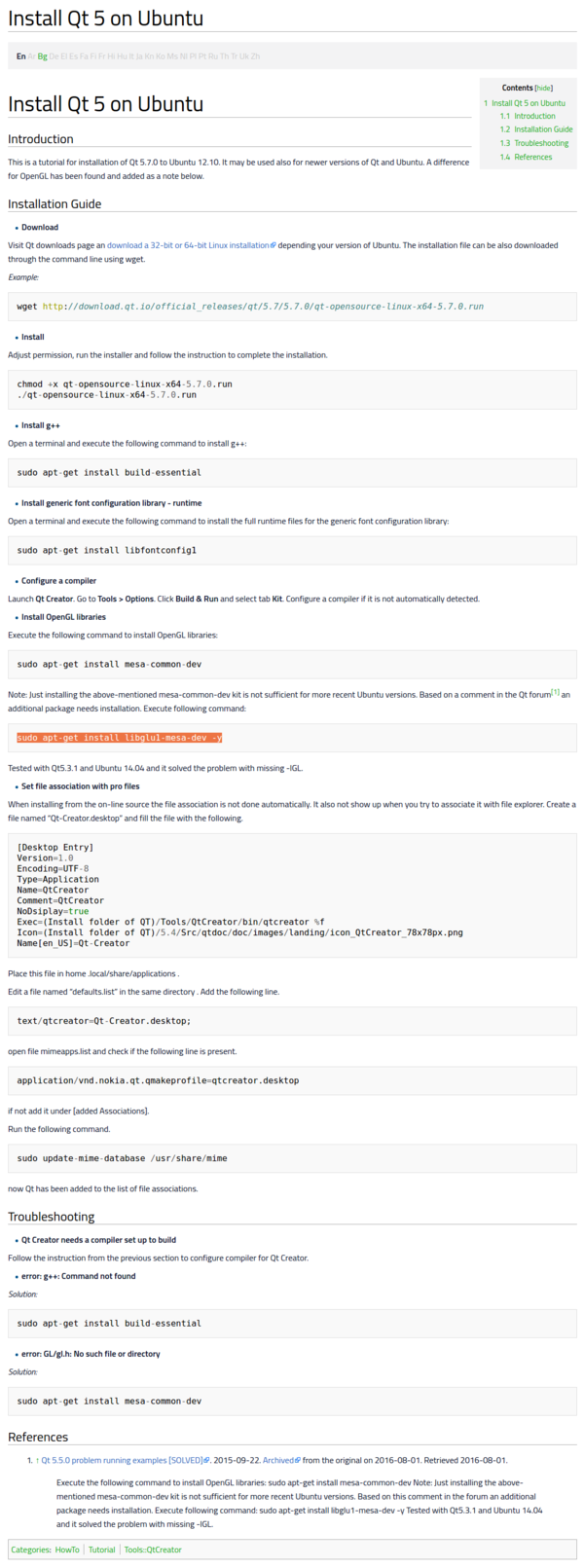
./20170807-1503-cet-1-17.png
- There is no difference on friend declaration, as long it is clearly stated which class friend to which class.
- There is global friend function/variable and then there is local friend function/variable as well.
- Here is an example to declare the whole class as a friend function.
friend class friend_class;
- Here is an example of global friend function.
friend void print_width (box _b);
- Here are my example codes for friend function/variable.
#include <iostream>
using namespace std;
// Prototype. Usually in box.h.
class box {
private:
double width;
public:
friend void print_width (box _b);
void set_width (double _w);
};
// Function definition usually in box.cpp.
void box::set_width (double _w) {
/* `width` can be accessed because this function accessed after `box`
object */
width = _w;
}
// "Global" function accessing `box` due to `friend` function.
void print_width (box _b) {
// This function can access private variable of `box`.
cout << "width: " << _b.width << endl;
}
// Main function for the program.
int main () {
box b;
// Set box width with member function.
b.set_width(10.0);
// Use friend function to print the width.
print_width(b);
return 0;
}
- Use
typedef
to create an alias of type data or to group type data together.
- There is
template
as well but I am not sure what is the use of it.
- Some documentations about
typedef
and template
can be followed in here, http://en.cppreference.com/w/cpp/language/typedef and here http://en.cppreference.com/w/cpp/language/type_alias.
static
function and variable is used if you want to have class - wide global function and variable. So all class with the same name or children can access the "global" function/variable that is that static
function/variable.
- I can just declare a global variable without a class encapsulate it.